Android browser
In order for our activity to access the internet and load web pages in the WebView, we need to specify the Internet permission for our app in the AndroidManifest.xml file as follows:Hide Copy Code<uses-permission android:name="android.permission.INTERNET"/>
Following is the code of the AndroidManifest.xml file.Hide Shrink
Copy Code<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.azim" >
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
I have used the following nested Linear Layout to display TextView, EditView, Button and WebView controls.Hide Shrink
Copy Code<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/txtWebUrl"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Enter URL: " />
<EditText
android:id="@+id/edtWebUrl"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<Button
android:id="@+id/btnGo"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:onClick="showPage"
android:text="Go"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<WebView
android:id="@+id/myWebView"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
</LinearLayout>
The android:onClick
attribute of the Button view in the above code specifies that the showPage()
method is the event handler for the click event of the Go button.The loadUrl()
method of the WebView control is used to load a web page to be displayed. The setBuiltInZoomControls()
method of the WebSettings
class can be used to display the built-in zoom controls. The setJavaScriptEnabled()
method of the WebSettings
class can be used to enable JavaScript on the page. The following code snippet is used to load the home page of the Google web site as well as display the built-in zoom controls:Hide Copy Codeview=(WebView)findViewById(R.id.myWebView);
WebSettings settings=view.getSettings();
settings.setBuiltInZoomControls(true);
settings.setJavaScriptEnabled(true);
view.setWebViewClient(new Callback());
view.loadUrl("http://www.google.com");
In the above code, the setWebViewClient()
method prevents loading the URL in the browser of our device. We need to override the onKeyDown()
method of our activity in order to enable going back to the previous page in the history of the WebView. Following is the code of the onKeyDown()
method.Hide Copy Codepublic boolean onKeyDown(int keyCode, KeyEvent event)
{
if(keyCode==KeyEvent.KEYCODE_BACK && view.canGoBack())
{
view.goBack();
return true;
}
else
{
return super.onKeyDown(keyCode,event);
}
}
The following click event handler of the Go button displays the page whose URL is entered by the user on the EditText control.Hide Copy Codepublic void showPage(View v)
{
view.setWebViewClient(new Callback());
view.loadUrl(text.getText().toString());
}
Finally, we need to override the shouldOverrideUrlLoading()
method of the WebViewClient
class as follows to prevent the site being opened in the browser of our device.Hide Copy Codeclass Callback extends WebViewClient
{
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url)
{
return false;
}
}
Following is the output of execution of the app.
If we have an HTML file called Hello.html stored in the assets folder of the project, it can be opened using the following URL:
file:///android_asset/Hello.html

<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.azim" >
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name=".MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>

android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/txtWebUrl"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Enter URL: " />
<EditText
android:id="@+id/edtWebUrl"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<Button
android:id="@+id/btnGo"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:onClick="showPage"
android:text="Go"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<WebView
android:id="@+id/myWebView"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
</LinearLayout>
android:onClick
attribute of the Button view in the above code specifies that the showPage()
method is the event handler for the click event of the Go button.loadUrl()
method of the WebView control is used to load a web page to be displayed. The setBuiltInZoomControls()
method of the WebSettings
class can be used to display the built-in zoom controls. The setJavaScriptEnabled()
method of the WebSettings
class can be used to enable JavaScript on the page. The following code snippet is used to load the home page of the Google web site as well as display the built-in zoom controls:WebSettings settings=view.getSettings();
settings.setBuiltInZoomControls(true);
settings.setJavaScriptEnabled(true);
view.setWebViewClient(new Callback());
view.loadUrl("http://www.google.com");
setWebViewClient()
method prevents loading the URL in the browser of our device. We need to override the onKeyDown()
method of our activity in order to enable going back to the previous page in the history of the WebView. Following is the code of the onKeyDown()
method.{
if(keyCode==KeyEvent.KEYCODE_BACK && view.canGoBack())
{
view.goBack();
return true;
}
else
{
return super.onKeyDown(keyCode,event);
}
}
{
view.setWebViewClient(new Callback());
view.loadUrl(text.getText().toString());
}
shouldOverrideUrlLoading()
method of the WebViewClient
class as follows to prevent the site being opened in the browser of our device.{
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url)
{
return false;
}
}
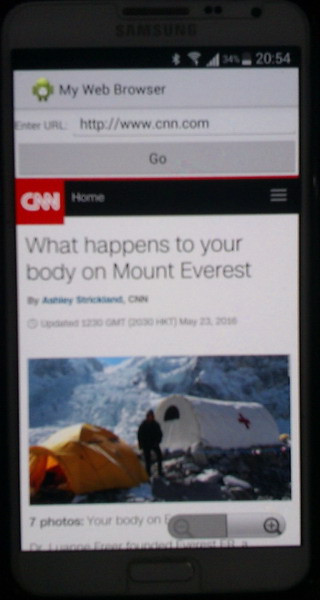
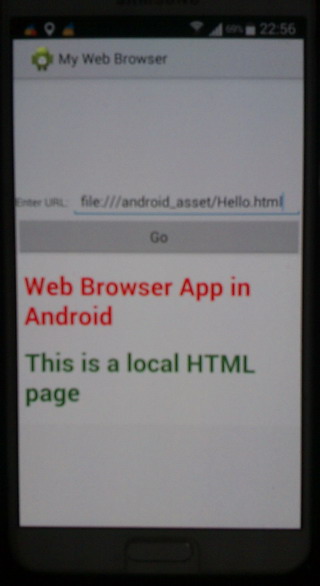
file:///android_asset/Hello.html
0 Comments: